Invoke Lambda method using end-user business wallet(Zero-Friction and Gasless)
In the last few tutorials, we discussed the powerful Metakeep Business Wallet
to batch transactions
and make payments
using your developer Business wallet. In this tutorial, we will discuss using end-user Business Wallets. The Lamba Invocation for end-user Business Wallets works differently and leverages the MetaKeep SDK to support gasless transactions for non-custodial end-user Business Wallets.
End-user Business Wallets are non-custodial
Only the wallet owner can perform operations on the wallet. MetaKeep and developers can't touch user Business Wallets.
In this tutorial, like earlier, we will start with a basic Voting Smart contract from our GitHub repo.
You can find an end-to-end working demo here.
Generate Consent Token
Consent token generation happens in the backend.
Consent token generation requires your API key. Never expose your secrets/keys to the public.
When a lambda invocation is requested on behalf of a user, you get back a Consent Token
- looks like a base64 string. This needs to be signed by the user before the invocation can happen. The token also gives MetaKeep the approval to pay for the transaction gas fees and bill you(the developer) later.
Users don't pay gas fee when using Business Wallets.
MetaKeep provides zero-friction gasless lambda invocations. However, if there is a
payment(aka value transfer)
such as transfering Ether from Business wallet, then the user is required to have the sufficient amount in their Business Wallet for the payment.
Behind the scenes, Consent Token is a cryptographic challenge generated directly by MetaKeep's hardware root of trust, based on non-custodial Private Key Management (ncPKM) infra, which can be satisfied exclusively by the cryptographic proof of identity of the end-user.
Step 1: Create a POST request to the API
To use business wallets, you need to send BUSINESS_WALLET
in the using
parameter of the lambda invocation. And then, specify the user as in the as
paramter.
To call a single lambda contract, use the Invoke Lambda API. Here's what the request looks like:
{
"lambda": "0x1234.....",
"function": {
"name": "function_name",
// keep it empty in case of no args
"args": [
"arg1",
"arg2"
]
},
"pay": "0.01",
"reason": "reason for invocation",
"as" : {
"email" : "<Email of User who will be invoking the lambda method.>"
}
"using": "BUSINESS_WALLET"
}
On the other hand, if you are looking to make multiple calls and payments, then use the Invoke Lambda (Batch) API. Here's what the request looks like:
{
"invocations": [
// First call with payment
{
"call": {
"lambda": "0x1234....",
"function": {
"name": "function_name",
"args": [
"arg1",
"arg2"
]
},
"pay": "0.01",
"reason": "reason for invocation"
}
},
// Second call with payment
{
"call": {
"lambda": "0xab12....",
"function": {
"name": "function_name1",
"args": [
"arg3",
"arg4"
]
},
"pay": "0.02",
"reason": "reason for this invocation"
}
},
// Payment to an address
{
"pay": {
"to": {
"ethAddress": "0xadd...."
},
"amount": "1.11"
}
}
],
"reason": "high level reason for the batch",
"as" : {
"email" : "<Email of User who will be invoking the lambda method.>"
}
"using": "BUSINESS_WALLET"
}
Step 2: Get the User's Approval
On making the API call, you will a consentToken
back in the response.
{
"status": "USER_CONSENT_NEEDED",
"consentToken": "CrgBAQIDAHjkSP_5liFU1kRiwcsjaYhL70lMH28zlSAGMUGu-qcyhwFlzokiZCBBJXIGos8D3l02AAAAfjB8BgkqhkiG9w0BBwagbzBtAgEAMGgGCSqGSIb3DQEHATAeBglghkgBZQMEAS4wEQQMFqkScqTlO68uvf6-AgEQgDvbTbd4sVsiGC9kpvrQcvRdl0LMFiVmg0K0eHZnlYm1M1mMyOV4X99ONhwBF3DHbtEcHs_Hfpg9AtHdExK1AgoM6uVs1U4BA3NKsVb5EqQCOPEYB6rgp_v5U-K71Rm7g1bbPhLKchRonB2teGsr7mi3tsENX0pm4fRn8al8EbeHmiSQCtvK-qAFr6SMYHW9wkc3_HBmrYYz6NbZpmie8xn3UllEy7YyWHEh7nRCajXKF79j3k-DOsdoWUe58ML_blzpicwcsNB358A7pIe6-hBn4XNL5wll_UXJEQBmDAPLp650CXmINwJWawWnQo3Jj4neTtNzXepLIWmJXRX1oW-hMyYq6Ng5oWxdkuCTqRnhRTxD5esADlbzArq8RZkM4lcPX2uNhcxAvMVS9sEhTVeFHTsuXKS-b1y-e7g5FKfe-5_lDdN-tX76LttLBWAbp05HFv5cXsAMDvI4y7_zVDQ0zDmKu4InZ_zgfJuwTh5faX98Sg=="
}
Once you have the consentToken
, use the MetaKeep SDK to get the user's approval for Lambda invocation.
const consent = await sdk.getConsent(consentToken);
Running the Demo Application.
You can find an end-to-end working demo here. Follow these steps to get the demo running:
Step 1: Prerequisite
Before proceeding, you must finish the first part of the tutorial Create Lambda. Once you have the Lambda contract address, store it somewhere because we will need that later.
Also, make sure that end-user Business Wallet has sufficient tokens for the payment(value transfer)
.
Step 2: Update the .env file.
Update the .env file with the API key and the Lambda address you got in the first step.
Step 3: Start the backend server.
Navigate to the lambda/business-wallet/batch-transactions/backend
directory. Then, start the backend server.
npm install
npm run start
This will start the server at port number 3001. This server will allow you to create proposals, vote, and make payments.
Step 4: Start the frontend server
Open a new terminal window, navigate to the lambda/business-wallet/batch-transactions/frontend
directory, and run the following command.
npm install
npm run start
This will open the demo application in your browser.
Step 5: Create a New Proposal
Before you ask users to vote, first create a proposal, else, the vote will fail.
Tap the Add Proposal
button in the header and add the details like proposal name and proposal description.
Ideally, in production, only your admins will have access to this page.
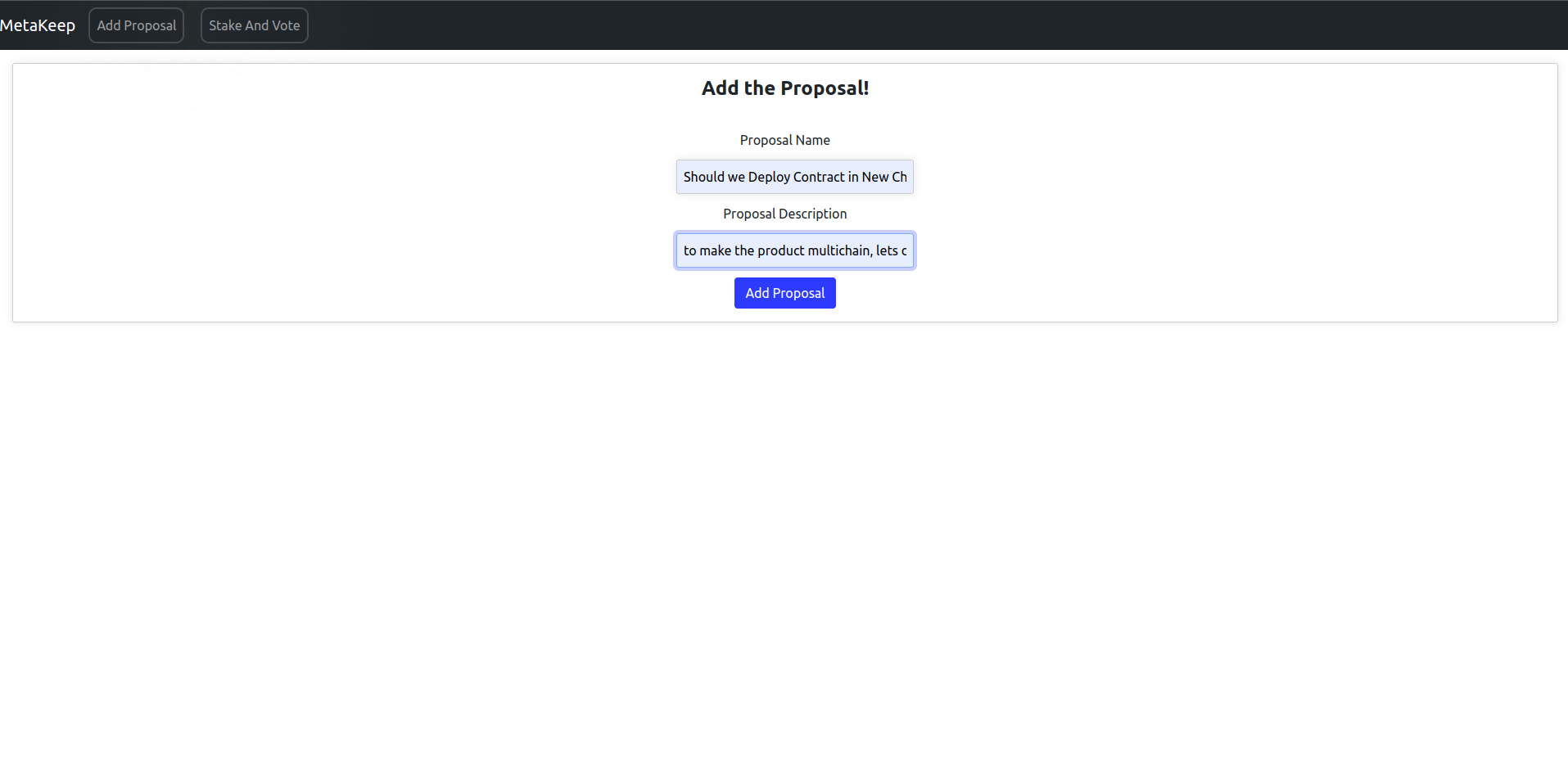
After you click Add Proposal
MetaKeep will add the transaction to the queue.
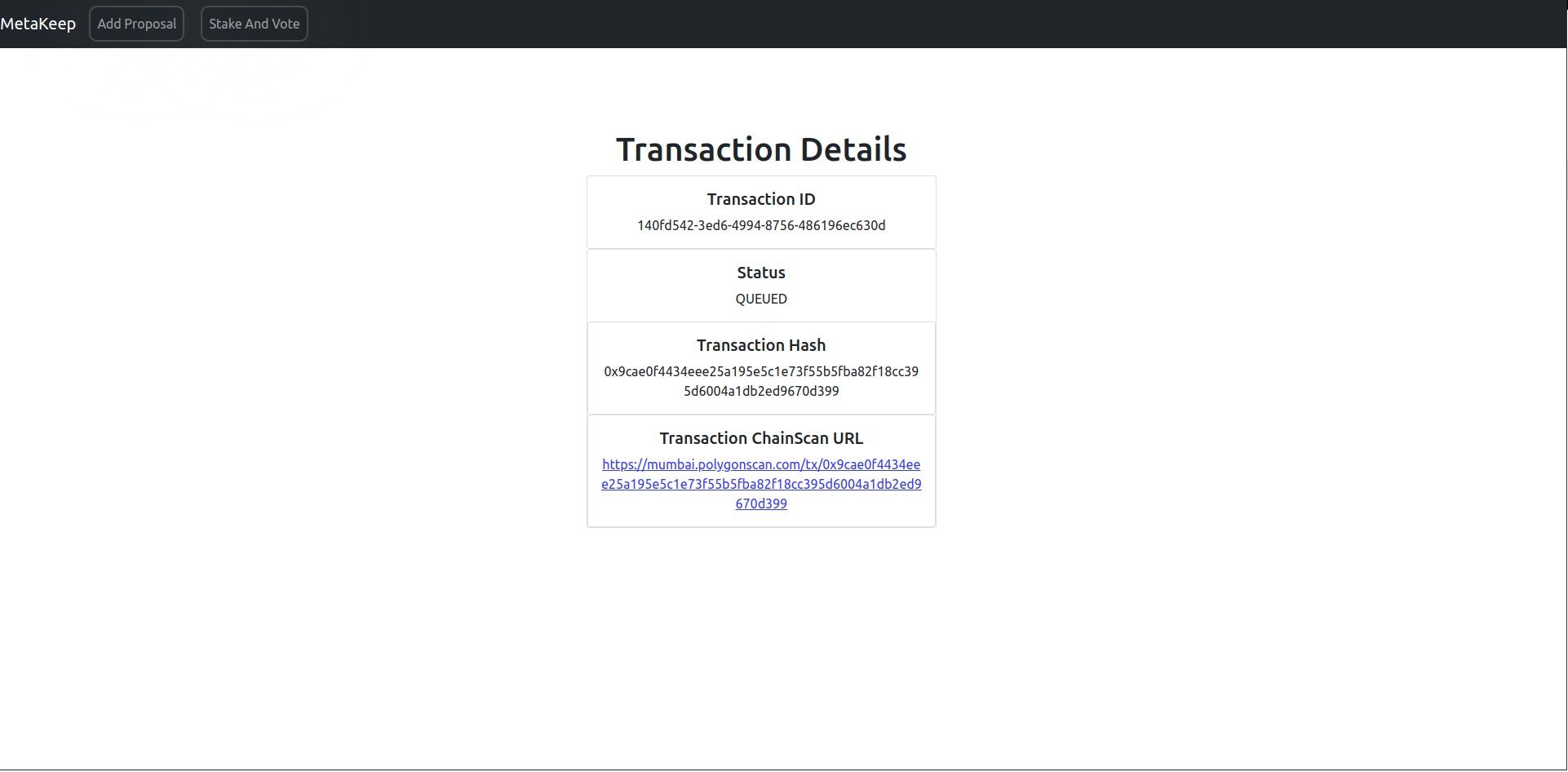
Ensure that the transaction is successful using MetaKeep's Transaction Status API or checking on the Transaction Chain Scan URL
.
Step 6: Get the Details of the Proposal.
Tap the Stake And Vote
button in the header, enter the Proposal ID
, and tap the Get Proposal
button.
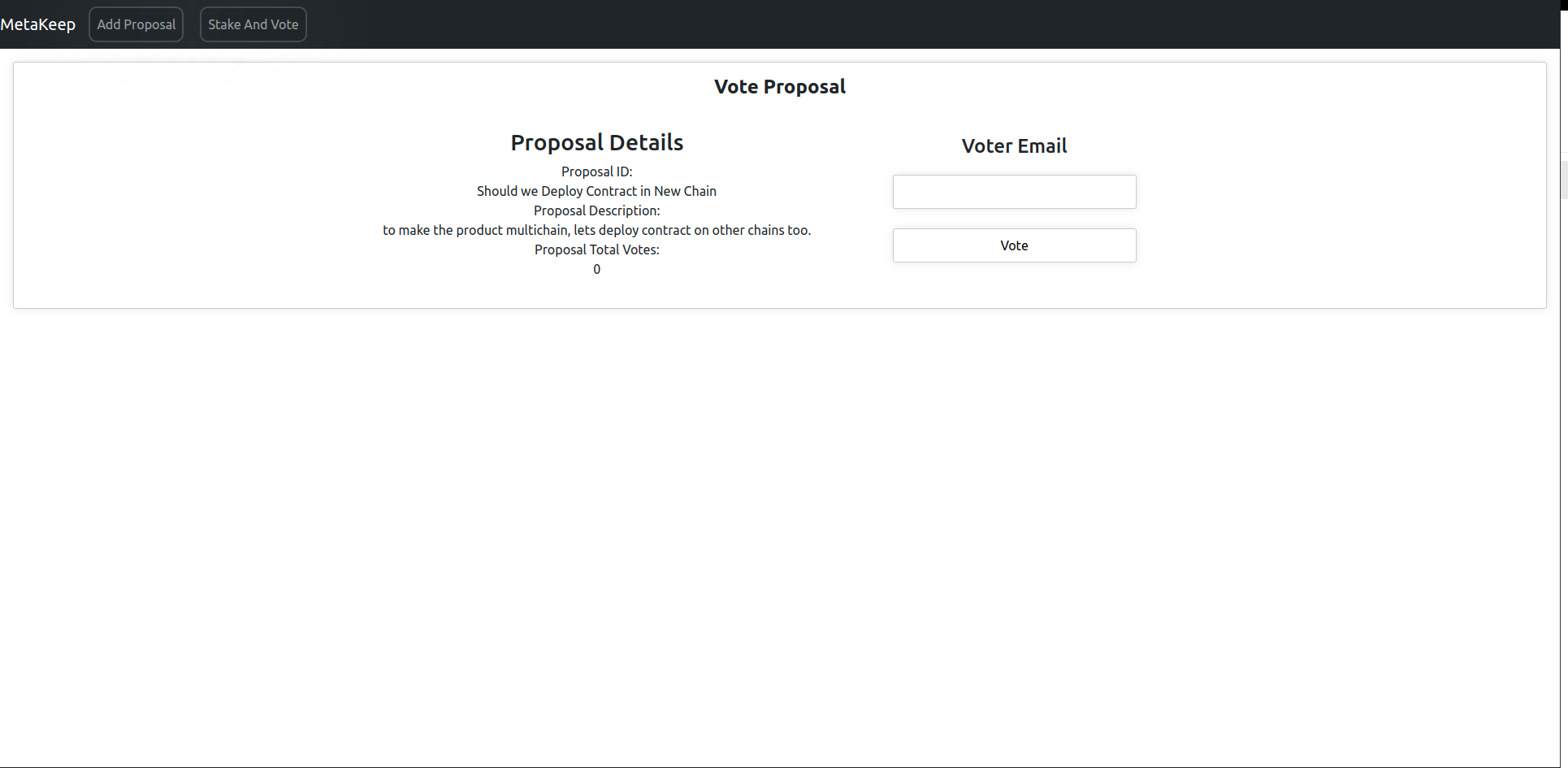
You should be able to see the proposal details, the number of votes, and an option to enter the voter's email.
Step 7: Enter the email from which you want to vote and click vote.
The backend will generate a Consent Token
and the frontend will ask for the user's approval using the MetaKeep SDK. Notice the batch invocation to vote for the proposal.
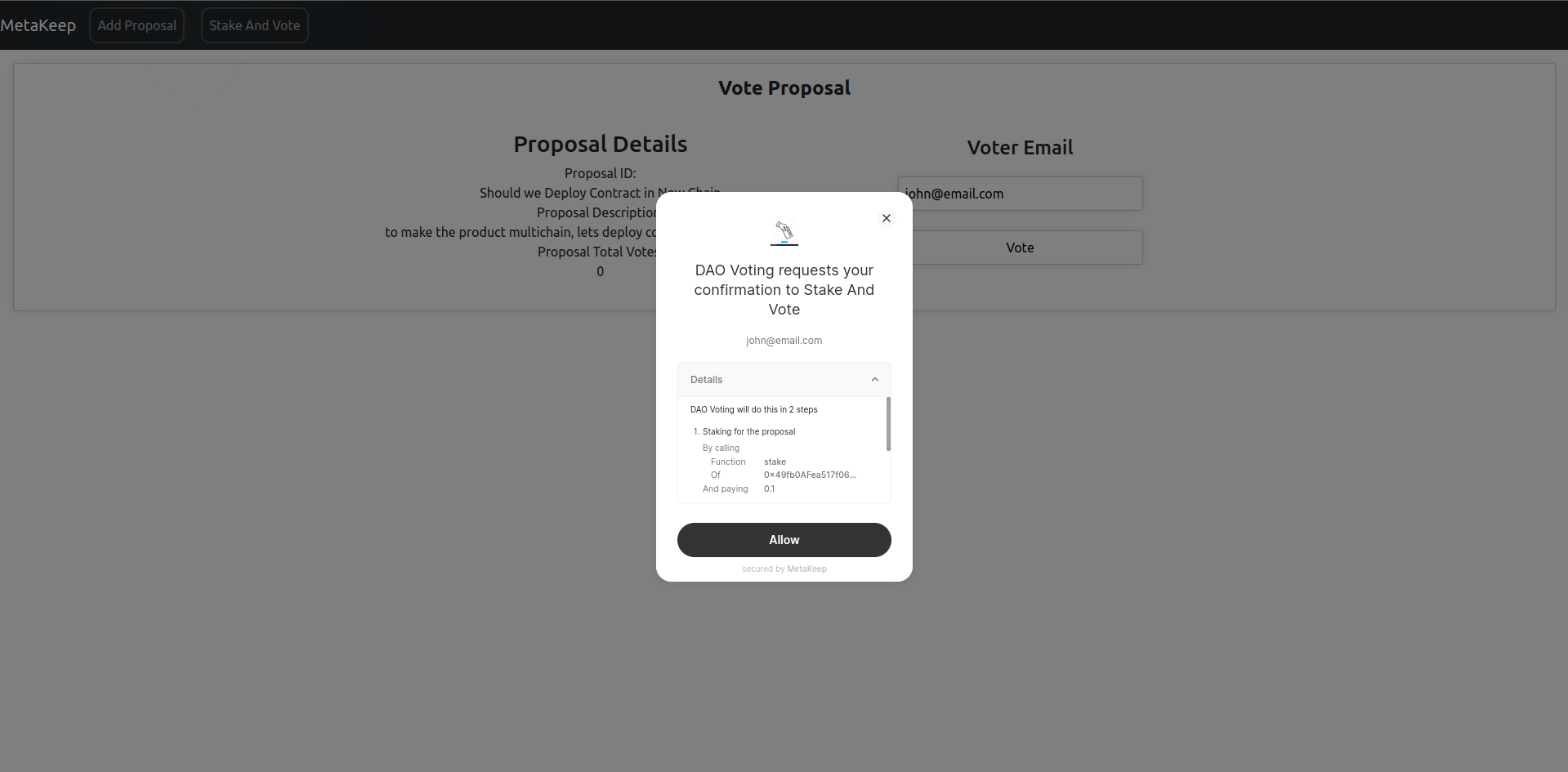
Step 8: Approve the Vote.
For verification, MetaKeep will send an OTP to the user's email. Copy the OTP and paste it into the approval UI.
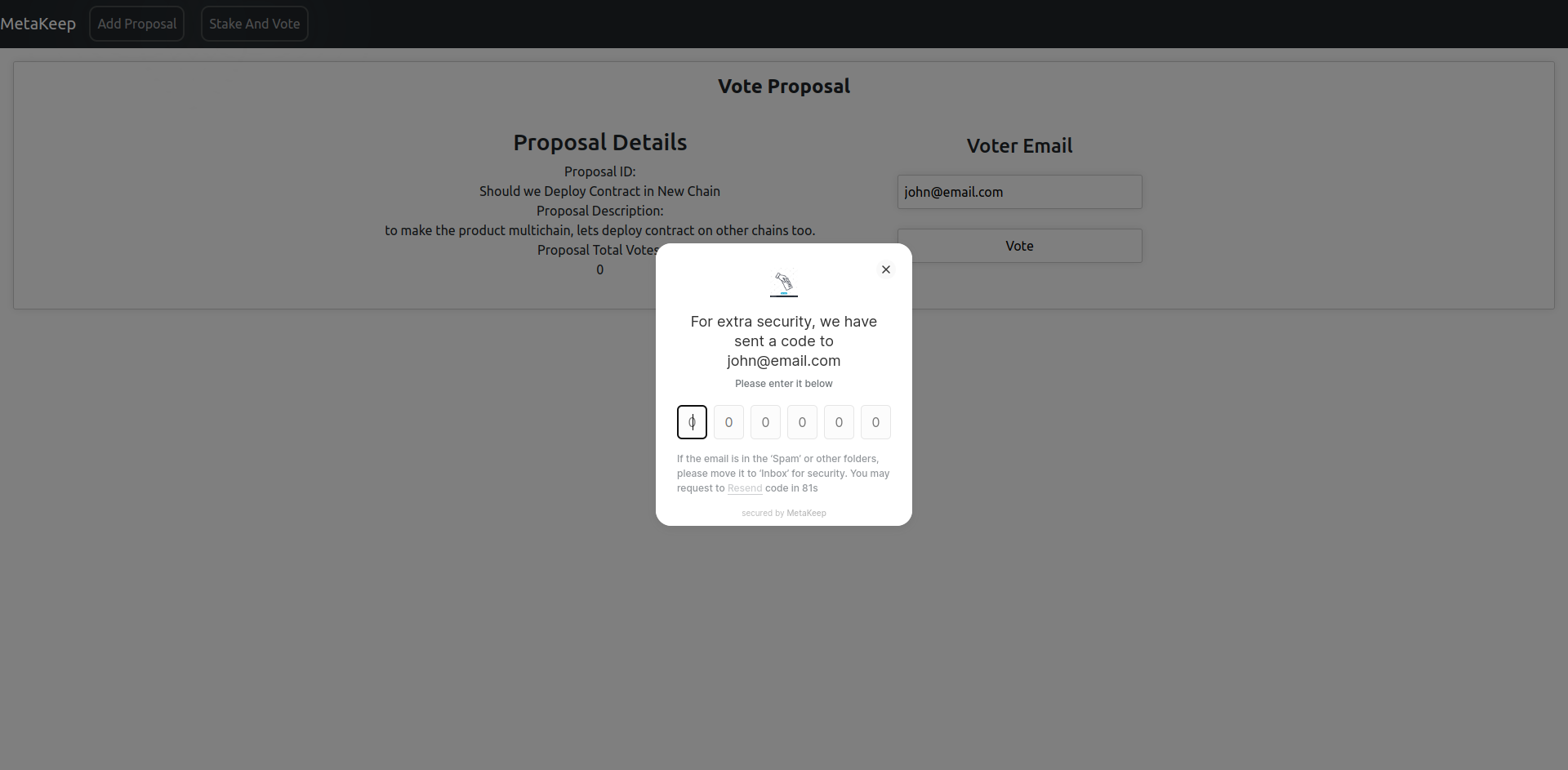
Once the verification succeeds, MetaKeep will queue a new transaction to the blockchain.
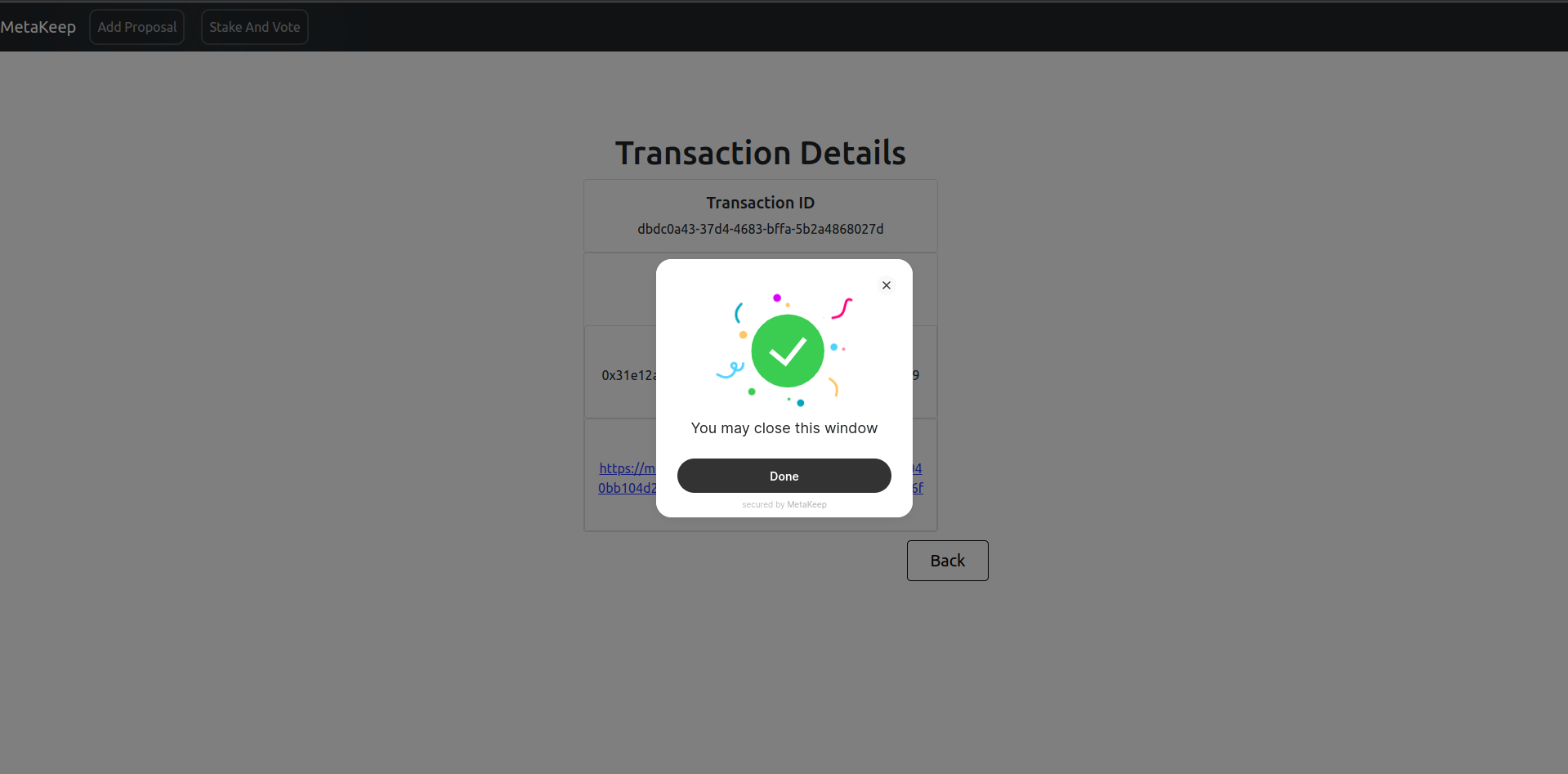
After you close the window, you can view the transaction details like below.
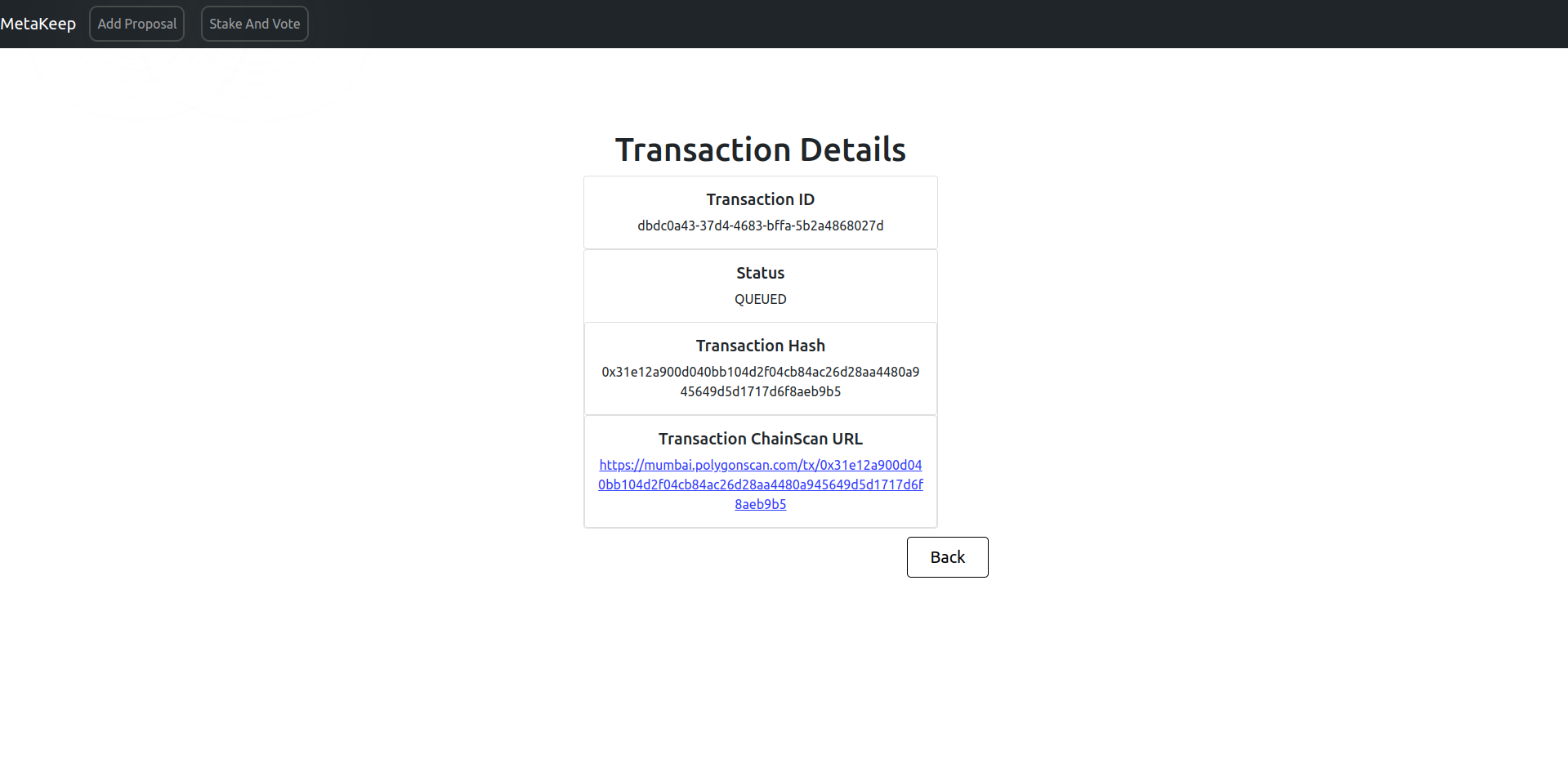
MetaKeep, behind the scenes, employs several strategies to ensure transaction succeeds and at the lowest cost. To get your transaction's status, you can also use the Transaction Status API.
Next Steps
Congratulations🎉🎉🎉🎉!!!! You have now enabled zero-friction and gasless blockchain application transactions for your end users.
Updated about 1 year ago