MetaKeep React Native SDK is a thin and lightweight wrapper around MetaKeep Android and iOS SDKs. It is written in TypeScript and provides type definitions for all the methods. You can find an end-to-end working code sample here.
Requirements
The SDK requires a minimum Android API version 26 (Android 8.0) or higher and Java 8 or higher on Android.
This is how android/build.gradle
file should look like:
buildscript {
ext {
//...
//---> Specify minimum API version
minSdkVersion = 26
//<---
//...
}
}
On iOS, SDK requires iOS 14.0 or higher, Xcode 14.0 or higher, and Swift 5.0 or higher.
This is how ios/Podfile
file should look like:
# ....
platform :ios, '14.0'
# ....
Installation
Copy the MetaKeep React Native SDK package from here and copy it to your project's modules
folder.
Then, install the package using yarn
or npm
:
npm install ./modules/MetaKeepReactNativeSDK
Also, make sure to run pod install
after installing the SDK in the ios
folder of your project.
Configure Callback
Android
Define the manifest placeholders for the MetaKeep Domain and Scheme. MetaKeep uses this to register an intent-filter
for your app and send data back to your app after the user operation.
Define the following placeholders in your android/app/build.gradle
file:
- metakeepDomain: This should be
<app_id>.auth.metakeep.xyz
where<app_id>
is your app's ID which you can find in the developer console. - metakeepScheme: This should be your app's package name.
This is how your app's updated android/app/build.gradle
file should look like:
android {
compileSdkVersion 30
defaultConfig {
//...
//---> Configure MetaKeep Domain and Scheme
// metakeepDomain: Replace <app_id> with your app's id which you can find in the developer console
// metakeepScheme: Replace com.domain.app with your app's package name
manifestPlaceholders = [metakeepDomain: "<app_id>.auth.metakeep.xyz", metakeepScheme: "com.domain.app"]
//<---
}
//...
}
iOS
MetaKeep uses a custom URL scheme to send data back to your iOS app after the user's operation.
Navigate to the Info tab
of your app target settings in XCode. In the URL Types
section, click the +
button to add a new URL. Enter the following values:
- Identifier: metakeep
- URL Schemes: $(PRODUCT_BUNDLE_IDENTIFIER)
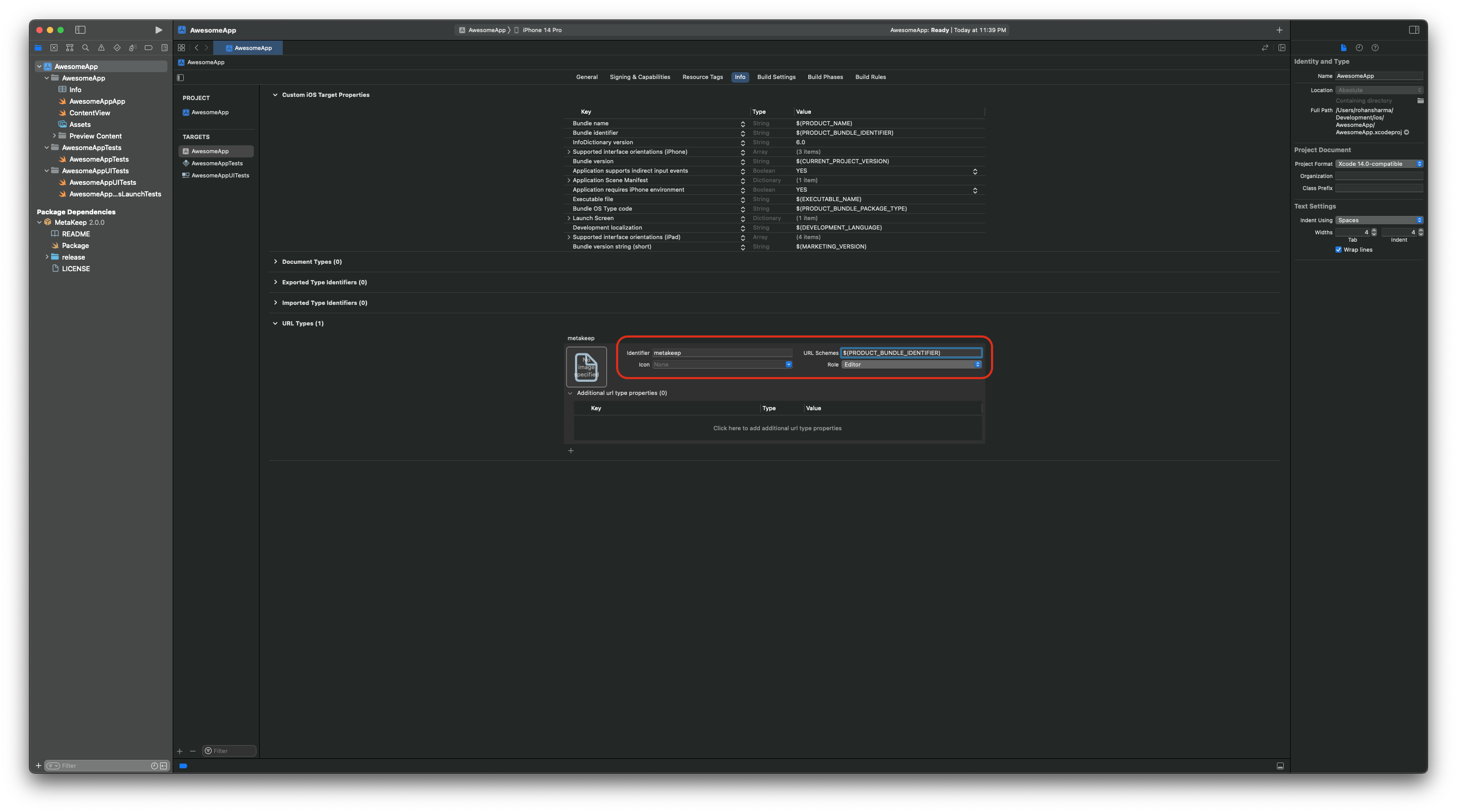
Create new URL Type
Then, configure your app to handle the callback URL. The URL is invoked by MetaKeep after the user's operation. The URL contains the result of the operation.
To capture the callback URL, add the following code to your ios/<YourAppName>/AppDelegate.mm
file:
#import <MetaKeep/MetaKeep.h>
// Setup MetaKeep callback handler
- (BOOL)application:(UIApplication *)application
openURL:(NSURL *)url
options:
(NSDictionary<UIApplicationOpenURLOptionsKey, id> *)options {
// Allow MetaKeep to handle the deep link URL.
// If you are handling other deeplinks, you may want to add some logic
// to only do this if the URL's format matches the one used by MetaKeep.
[[MetaKeepMetaKeepCompanion companion] resumeUrl:url.description];
return true;
}
© Copyright 2024, Passbird Research Inc.